Unity Character Control with Keyboard
I will show you 2 ways to move your character with keyboard.
Unity Hello World
When you open Unity 3D, you will see a window like this. Under the “Hierarchy” title, a main camera is created as default and you can create your objects in here and see it in “Scene” window. In Scene window, you can move, rotate and resize your objects. When you select an object, its specifications will be shown in “Inspector” window. And if you press play button, your game runs in “Game” window. “Project” window includes all materials of your project such as images, materials, prefabs, scripts etc. You can change layout style by the options at top-left. Are you ready to say “Hello World”?
Click “Create” button under project title and create a C# script. Rename it as “HelloWorld”. Double-click on it and “Mono Develop” will be open as default if you don’t use another compiler. Type
in Start function and save it. Back to Unity, drag HelloWorld script into the Main camera and press play. You will see our text in “Console” window. Functional scripts should be attached in a game object in scene to be used.
Click “Create” button under project title and create a C# script. Rename it as “HelloWorld”. Double-click on it and “Mono Develop” will be open as default if you don’t use another compiler. Type
Debug.Log("Hello World");
in Start function and save it. Back to Unity, drag HelloWorld script into the Main camera and press play. You will see our text in “Console” window. Functional scripts should be attached in a game object in scene to be used.
Unity Tile-Based Ground
Tile-Based Ground – Unity3D (C#)
To create a tile-based ground, we need a simple matrix system. A (row X column) matrix will take a plane texture and draw it in each cell.
To create a tile-based ground, we need a simple matrix system. A (row X column) matrix will take a plane texture and draw it in each cell.
public GameObject plane; public int width = 10; public int height = 10; private GameObject[,] grid = new GameObject[30, 30]; void Start () { for (int x =0; x < width; x++) { for (int y =0; y < height; y++){ GameObject gridPlane = (GameObject)Instantiate(plane); gridPlane.transform.position = new Vector2(gridPlane.transform.position.x + x, gridPlane.transform.position.y + y); grid[x, y] = gridPlane; } } }
Tile-Based Ground – Unity3D (C#)
Unity Grid System
Grid System – Unity3D (C#)
Grid system is used mostly in RTS games. Your characters or buildings move on grids. You need a matrix to create a grid system
Grid system is used mostly in RTS games. Your characters or buildings move on grids. You need a matrix to create a grid system
public float cell_size = 2.0f; private float x, y, z; void Start() { x = 0f; y = 0f; z = 0f; } void Update () { x = Mathf.Round(transform.position.x / cell_size) * cell_size; y = Mathf.Round(transform.position.y / cell_size) * cell_size; z = transform.position.z; transform.position = new Vector3(x, y, z); }
Grid System – Unity3D (C#)
Draw grid on the terrain in Unity
Destroyable terrain trees in Unity
How to Create Simple Timer
Unity How to Create Simple Timer C#
A visible or background timer runs on most games. We will create an easy one which ticks seconds. In this tutorial we don’t use any value such as integer, float etc. We just need a GUI text. Let’s create.
Open your project and create a GUIText. Right-Click on hierarchy window, UI/Text.
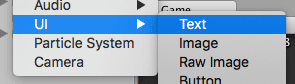
Edit its text. This step is important because we will use this text. Set it as your timer beginning value.
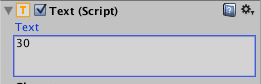
Now create a C# script. In this script, we are going to take the value of GUIText and decrease it by 1 every second. Don’t forget to implement using UnityEngine.UI; lib because Text needs it.
public class Timer : MonoBehaviour { public Text scoreText; void Start () { InvokeRepeating("RunTimer", 1, 1); } void RunTimer() { scoreText.text = (int.Parse(scoreText.text) - 1).ToString(); } }
That’s it. Set your UI text as scoreText and run it. We got a “seconds” timer with a simple logic.
WorldPress (WordPress JSON API + Unity3D + HTML5 / WEBGL)
Can WordPress power a Unity5 website? What would it be like to play a Unity game where the content is influenced and managed by a WordPress website… can we play with WordPress data? Could we construct a world around it?
You can display gizmos in the game window
Unity: You can display gizmos in the game window
You can display gizmos in the game window just like you can do in the scene window.
Use Layers popup to lock objects and control visibility
Unity Use Layers popup to lock objects and control visibility
By placing your objects on different layers you can control if these objects should be locked on your scene or not. It may be a good idea to lock layers that you don’t want to move by accident (like ground game object). In order to lock a layer, use the Layers popup that is located in the top-right corner of your Unity editor.
Use [SerializeField] and [Serializable] properties to control class serialization
Unity: Use [SerializeField] and [Serializable] properties to control class serialization
By default Unity serializes supported public fields and ignores all private fields. You can control that behavior using [SerializeField] and [Serializable] attributes.
[SerializeField] private int accumulator; // it will be serialized too!You can also do the opposite – having a public field, but its value shouldn’t be stored.
[NonSerialized] public float currentHealth;
If you want to create a custom inner type (inside another class) and serialize it inside it, you must add to it a [Serializable] attribute.
class World : MonoBehaviour { public Bot[] bots; [Serializable] public class Bot { public string name; public int health = 100; [SerializeField] // you can use it here too! private int somethingHidden = 5; } }
Please refer to the Unity3D documentation to read more about [SerializeField], its limitation, and possibilities.
Unity Reset Transform values with only two clicks
Unity Reset Transform values with only two clicks
When creating new Game Object its transform values are set in a way that you can see this new object in your Scene window. If you want it to be a container for other objects or this should be just an empty management object with scripts attached, it is strongly recommended to place it at local 0, 0, 0 with no rotation and the default scale.Use Game Object icons to clarify your scene view
Unity Use Game Object icons to clarify your scene view
For all your game objects you can define how it should look like in the scene view, even if this game object doesn’t have any renderer attached. For this purpose you can use nameless icon:
Use Export Package option to share your work
If you’re not working alone, you need to find a way to share your project files with other people. Unity project files consists on hidden (by default) meta files which are responsible for keeping references. This is the reason why copying your Assets folder won’t work at all. Unity comes with unitypackage format that preserves all references between files and can be freely shared.
Hold ALT while opening Unity to open Project Wizard
Low Poly Rocks Pack
Low Poly Rocks Pack - Free Download
Few day ago I posted several screenshots of my rocks and you guys liked it so much. Thanks.3 Unity Optimization Tips
3 Unity3D Optimization Tips
We are nearing the end of our current project, and as such I am starting to look into optimizations. What better time to share some little-known Unity facts with others than right now?
We are nearing the end of our current project, and as such I am starting to look into optimizations. What better time to share some little-known Unity facts with others than right now?
How To Add UI Text Outline
Unity3D How To Add UI Text Outline
I had a scene with a colorful background and some UI text over it. The problem was that the text was hard to read because of the lack of contrast between the text color and the background. So I added a UI text outline component to the Text object.Add Outline Component
Expand your canvas, select the Text object and go to the Inspector. Click the Add Component button and type in Outline, then click the result to add the Outline component.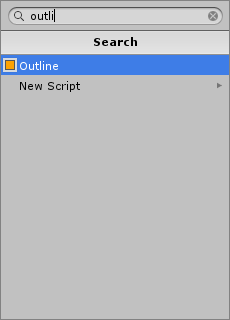
Customize Outline Component
The Outline component has only three features that you can edit. In my case, I had white text, black outline over light blue background. I left the default settings except I found that unchecking the “Use Graphic Alpha” helped a little.I also added some text shadow which helped as well. Check out my article on how to do this in Unity3D How To Add UI Text Shadow.
Android Back Button Best Practice
Unity3D Android Back Button Best Practice
Google has done extensive analysis and focus group research on Android user behavior. One important issue that Google research has identified for Android developers is to always have a listener for the Back button. It seems that Android users become angry or irritated if the application or game ignores the back button. In Unity3D simply add the following code into your loop:
if (Input.GetKeyUp("escape")) { // Quit application Application.Quit(); }
Unity3D How To Get Current Scene Name
Unity3D How To Get Current Scene Name
I created a simple countdown timer that jumped to the next scene. I wanted to reuse the same script for this effect, two times. Instead of rewriting the same script, I needed to detect the current scene name so the script knows where to jump next. Here is how:
I was confused for a sec that the .name was a member of GetActiveScene() but it was cleared up when I found an example on the Unity3D forum.
I created a simple countdown timer that jumped to the next scene. I wanted to reuse the same script for this effect, two times. Instead of rewriting the same script, I needed to detect the current scene name so the script knows where to jump next. Here is how:
Include The Namespace
Include the SceneManagement namespace in your project:using UnityEngine.SceneManagement;
Get The Current Scene Name
Using the Start function you can grab the current scene name when the scene starts:string currentScene; void Start() { currentScene = SceneManager.GetActiveScene().name; }
Load The Target Scene
Now that you have the current scene name, you can figure out what to do in your loop:if( currentScene == "Intro" ) { SceneManager.LoadScene("MainMenu"); } else { SceneManager.LoadScene("Intro"); }
I was confused for a sec that the .name was a member of GetActiveScene() but it was cleared up when I found an example on the Unity3D forum.
How To Add UI Text Shadow
Unity3D How To Add UI Text Shadow
I needed to make the contrast between some text and background graphic more distinct. Adding UI text shadow helped. Here is how:Add UI Text Shadow
Go to your Canvas, then the text object and then go to the Inspector. Now click the Add Component button and type in Shadow. Click to add the Shadow component.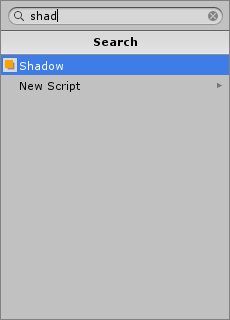
Edit UI Text Shadow
The UI Text Shadow component has a couple of features that you can edit. For my needs, I only changed the X offset from 1 to 2. This helped make the text contrast a little more distinct and easier to read in the game window.You can also add a text outline. See my article on Unity3D How To Add UI Text Outline.
Subscribe to:
Posts (Atom)