I will show you 2 ways to move your character with keyboard.
Create a cube named “Char” and create a script named “Movement”. You can attach this script to Char object but we won’t. Lets create an object before Start() function:
public GameObject character;
character is a public game object which means we can reach it from Unity manually. Now, write some if statements in Update() function. This fuction is called in runtime.
void Update () { if (Input.GetKey(KeyCode.RightArrow)){ Vector3 newPosition = character.transform.position; newPosition.x++; character.transform.position = newPosition; } if (Input.GetKey(KeyCode.LeftArrow)){ Vector3 newPosition = character.transform.position; newPosition.x--; character.transform.position = newPosition; } if (Input.GetKey(KeyCode.UpArrow)){ Vector3 newPosition = character.transform.position; newPosition.z++; character.transform.position = newPosition; } if (Input.GetKey(KeyCode.DownArrow)){ Vector3 newPosition = character.transform.position; newPosition.z--; character.transform.position -= newPosition; } }
Now save it and back to Unity. Attach to camera, select camera and you will see this script in inspector window. Drag Char object to Character bar in script and press play. Is it fast? Don’t worry, just a little change is needed. Back to code and add a float variable:
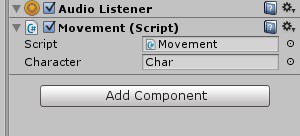
private float speed = 2.0f;
and replace if statements with:
void Update () { if (Input.GetKey(KeyCode.RightArrow)){ transform.position += Vector3.right * speed * Time.deltaTime; } if (Input.GetKey(KeyCode.LeftArrow)){ transform.position += Vector3.left* speed * Time.deltaTime; } if (Input.GetKey(KeyCode.UpArrow)){ transform.position += Vector3.forward * speed * Time.deltaTime; } if (Input.GetKey(KeyCode.DownArrow)){ transform.position += Vector3.back* speed * Time.deltaTime; } }
Current speed is 2.0f but you can change it.